API Single Integration Architecture
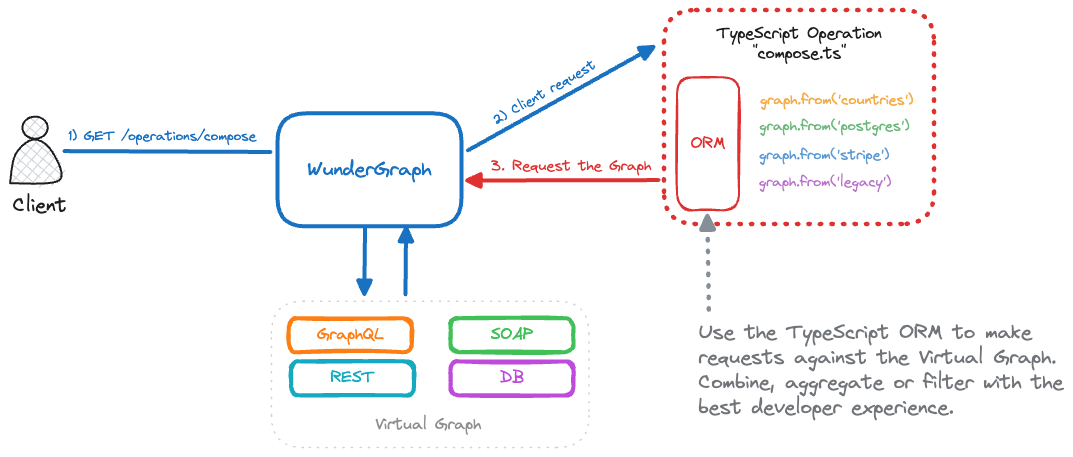
Problem
Managing Multiple Integrations and Complexity in External Systems
Developers often face the challenge of managing multiple integrations with external systems, which can lead to increased complexity and difficulty in maintaining connections. This complexity can result in a higher likelihood of errors and inefficient testing procedures. Single Integration Architecture (SIA) aims to solve this problem by providing a unified integration point with custom, type-safe logic, allowing for more efficient testing and fewer errors.
Solution
Implementing Single Integration Architecture
By implementing Single Integration Architecture, developers can maintain a single integration point, streamlining the development process and minimizing the potential for errors. However, there are some challenges that developers may encounter when using SIA.
Challenges with Single Integration Architecture
-
Code Complexity: Implementing SIA can introduce additional code complexity, as developers must manage various API configurations, operation definitions, and custom logic.
-
Boilerplate Code: SIA can generate a significant amount of boilerplate code, leading to increased development time and maintenance efforts.
-
Time-consuming API Configuration: Configuring and managing APIs within an SIA can be a time-consuming process, particularly when dealing with multiple external systems.
Overcoming Challenges with Centralized Systems like WunderGraph
Centralized systems such as WunderGraph simplify the implementation of Single Integration Architecture by reducing the burden of boilerplate code and streamlining API configuration and operation definitions. By leveraging WunderGraph's capabilities, developers can focus on building robust applications without worrying about the complexities associated with managing multiple integrations.
Example: Simplifying API Configuration with WunderGraph
Consider a scenario where a developer needs to integrate with multiple external systems, such as a CRM, an e-commerce platform, and a payment gateway. Using WunderGraph, the developer can define a single configuration file that includes all necessary API connections and operation definitions:
// wundergraph.config.ts
const federatedApi = introspect.federation({
apiNamespace: 'federated',
upstreams: [
{
url: 'http://localhost:4001/graphql',
},
{
url: 'http://localhost:4002/graphql',
},
],
});
const legacySoapAPI = introspect.soap({
apiNamespace: 'legacy',
source: {
kind: 'file',
filePath: './legacy.wsdl',
},
headers: (builder) =>
builder.addClientRequestHeader('X-Authorization', 'Authorization').addStaticHeader('X-Static', 'Static'),
});
const crm = introspect.openApiV2({
apiNamespace: 'crm',
source: {
kind: 'file',
filePath: './crm.json',
},
introspection: {
pollingIntervalSeconds: 2,
},
requestTimeoutSeconds: 10, // optional
});
const db = introspect.prisma({
apiNamespace: 'prisma',
prismaFilePath: 'path/to/prisma/schema.prisma',
});
configureWunderGraphApplication({
apis: [federatedApi, soap, crm, db],
});
Using WunderGraph, you will get a fluid API Orm that you can use to query your API's in a type-safe manner:
import { createOperation } from "../../generated/wundergraph.factory";
export default createOperation.query({
handler: async ({ graph }) => {
const company = await graph
.from('legacy')
.query('companies')
.where({ ticker: 'WMT' })
.exec();
const contacts = await graph
.from('crm')
.query('getContactsByLegacyCompanyId')
.where({ legacyId: company.id || '' })
.select(
'customer.name',
'customer.email',
'customer.company.icon',
'customer.company.description',
'customer.company.title',
).exec();
return {
company,
contacts,
}
}
});
In this example, WunderGraph (opens in a new tab) simplifies the API configuration process by allowing developers to define all external system connections within a single configuration file. This approach reduces code complexity and streamlines the development process.
Conclusion
Implementing Single Integration Architecture using centralized systems like WunderGraph (opens in a new tab) can help developers overcome common challenges associated with managing multiple integrations and external systems. By leveraging WunderGraph's capabilities, developers can reduce code complexity, minimize boilerplate code, and streamline API configuration, resulting in more efficient testing and fewer errors.